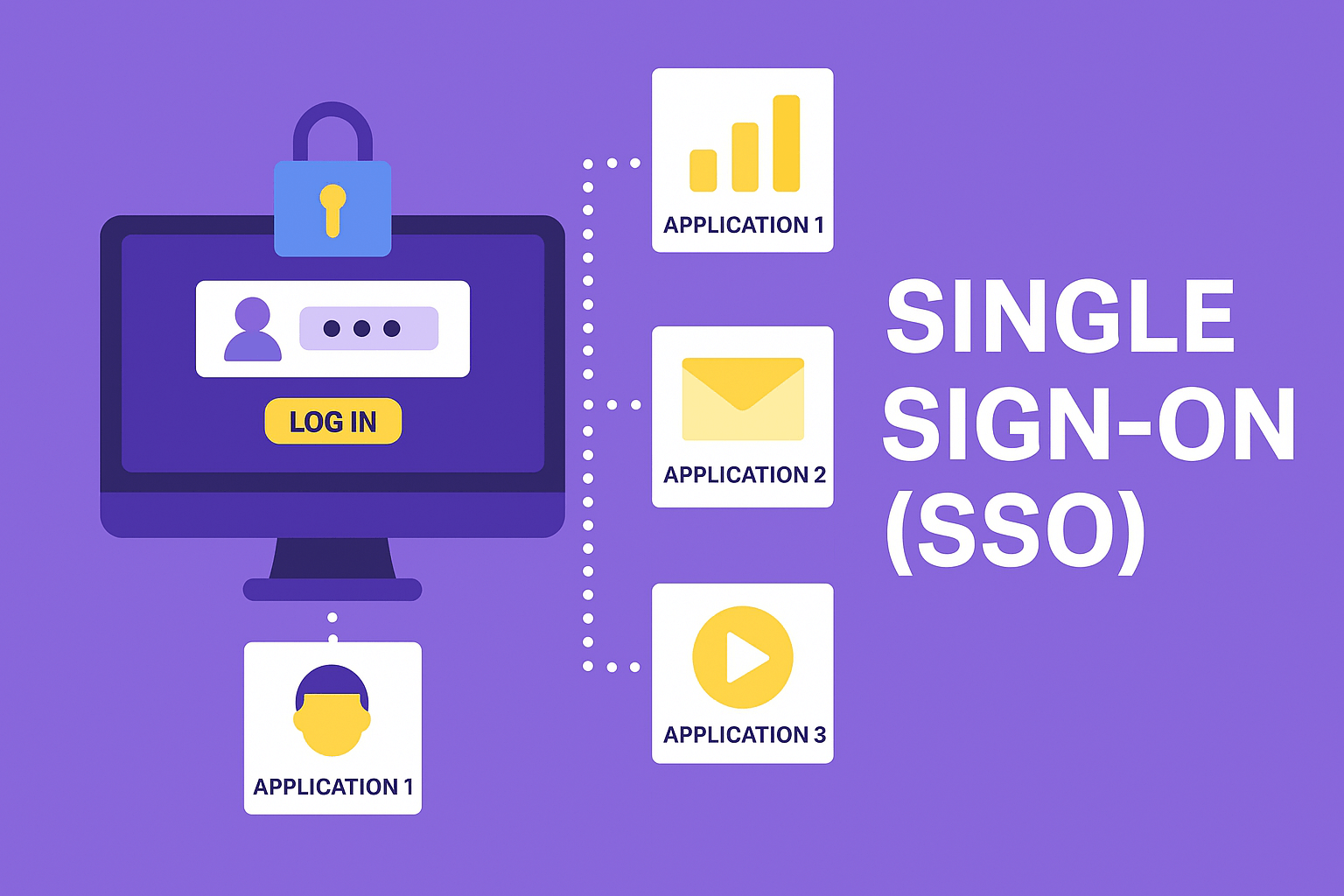
We have a requirement in our project that we have to restrict the api call to a limited number of call in a specific timeline.
How we can achieve this?
We have a requirement in our project that we have to restrict the api call to a limited number of call in a specific timeline.
How we can achieve this?
I try to implement the changes in the http interceptor but not able to achieve
I search some of the solutions, Not sure which one we have to implement
High Urgency
Intermediate
Implementing API rate limiting in Angular involves controlling the frequency of API calls to prevent abuse and ensure that your application stays within specified limits.
Here’s a basic approach to achieve this using Angular Interceptors.
Make the below changes in your Angular HTTP interceptor that will be responsible for rate-limiting API calls.
// rate-limit.interceptor.ts import { Injectable } from '@angular/core'; import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent, HttpResponse } from '@angular/common/http'; import { Observable, of } from 'rxjs'; import { tap } from 'rxjs/operators'; @Injectable() export class RateLimitInterceptor implements HttpInterceptor { private requestTimestamps: Map<string, number> = new Map<string, number>(); private maxRequestsPerInterval = 5; // Adjust as needed private intervalDuration = 1000; // Adjust as needed (in milliseconds) intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> { const url = request.url; // Check if the URL is subject to rate limiting if (this.shouldLimit(url)) { const lastTimestamp = this.requestTimestamps.get(url) || 0; const currentTime = new Date().getTime(); // Calculate the time elapsed since the last request const elapsed = currentTime - lastTimestamp; if (elapsed < this.intervalDuration) { // Rate limit exceeded console.warn(`Rate limit exceeded for ${url}`); return of(new HttpResponse({ status: 429, statusText: 'Rate Limit Exceeded' })); } } // Continue with the request return next.handle(request).pipe( tap((event) => { if (event instanceof HttpResponse) { // Update the timestamp for successful requests this.requestTimestamps.set(url, new Date().getTime()); } }) ); } private shouldLimit(url: string): boolean { // Add conditions to check if the URL should be subject to rate limiting return true; // Implement your own logic here } }
Include the interceptor in your Angular module providers.
// app.module.ts import { NgModule } from '@angular/core'; import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http'; import { RateLimitInterceptor } from './path/to/rate-limit.interceptor'; @NgModule({ imports: [HttpClientModule], providers: [ { provide: HTTP_INTERCEPTORS, useClass: RateLimitInterceptor, multi: true, }, ], }) export class AppModule {}
Modify the maxRequestsPerInterval and intervalDuration in the RateLimitInterceptor according to your rate-limiting requirements.
This basic example uses an in-memory Map to store the timestamps of the last requests for each URL and checks if the rate limit is exceeded. Adjust the conditions and data structures according to your specific use case.
Remember that this client-side rate limiting is not foolproof, as clients can manipulate or bypass it. For more robust rate limiting, consider implementing rate limiting on the server-side as well.
Got a question? Ask our extensive community for help. Submit Your Question
ionic, firebase, mobile app