What is SSO (Single Sign-On) and How Does It Work?
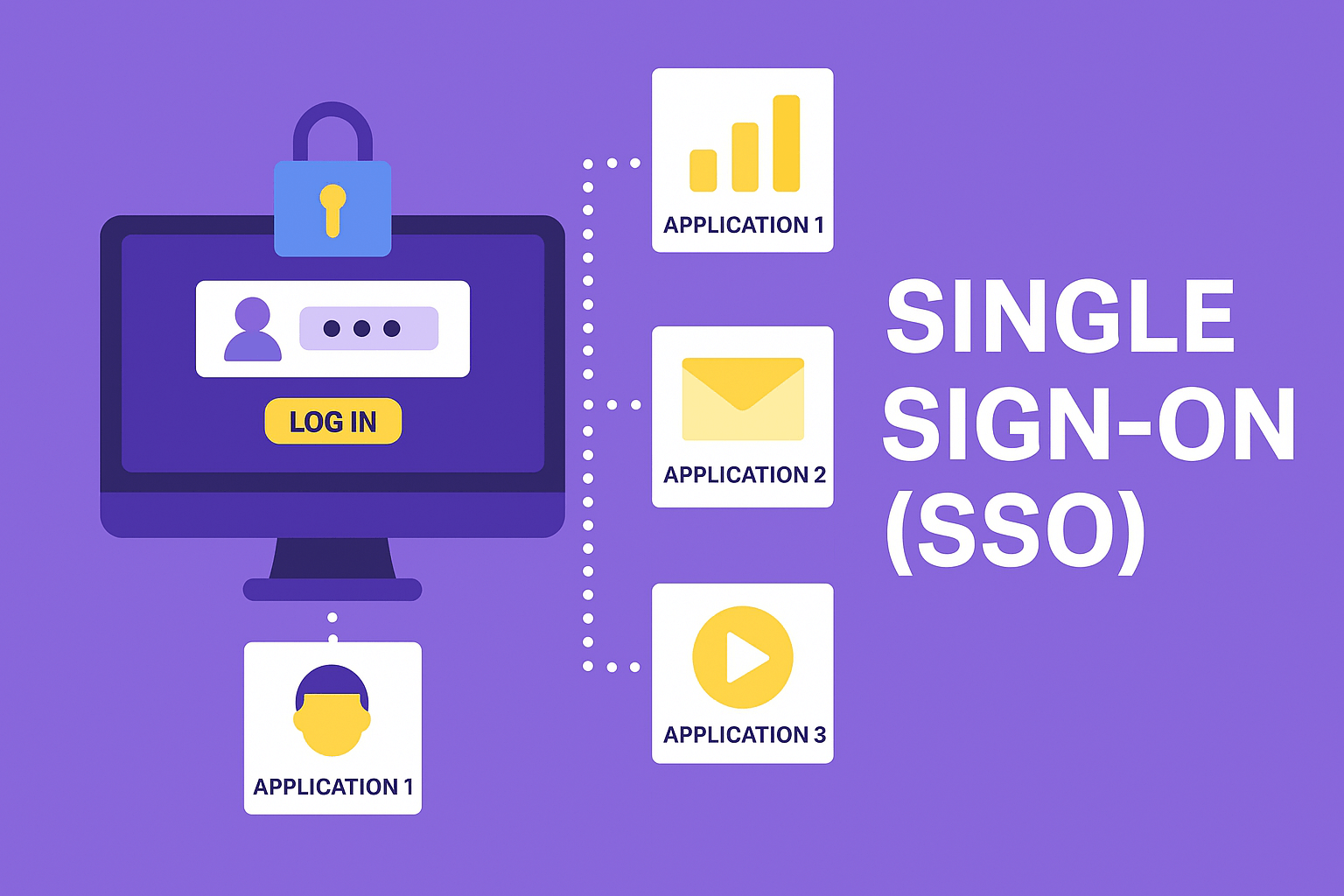
In today's digital world, logging into multiple platforms, apps, and tools has become a routine task. But let’s be honest—constantly entering usernames and passwords is annoying and inefficient. That’s where Single Sign-On (SSO) comes in. It’s a smart solution to a frustrating problem: login once and access everything. In this blog, we’ll break down what SSO is, how it works, its benefits, real-world examples, and even walk through a basic code snippet.
What is SSO (Single Sign-On)?
Single Sign-On (SSO) is an authentication scheme that allows users to log in once and gain access to multiple applications or systems without needing to log in again at each one.
In simple words: One password. Multiple services. Instant access.
Benefits of SSO:
- Time-saving: One login for all applications.
- User experience: Seamless transitions between services.
- Security: Reduces password fatigue and entry points for attacks.
- Centralized management: Easier control for admins.
How SSO Works – A Step-by-Step Example
Let’s use a practical, real-world scenario with Google services like Gmail and YouTube.
🔹 Step 1: User visits Gmail
- The user tries to access Gmail.
- Gmail notices the user is not logged in and redirects them to the SSO authentication server.
🔹 Step 2-3: User logs in at SSO login page
- The SSO server also sees the user is not logged in, so it displays a login page.
- User enters credentials.
- The server validates the credentials, creates a session, and generates a token.
🔹 Step 4-7: Gmail receives token
- Gmail sends the token to the SSO server for verification.
- The SSO server verifies and responds with "valid".
- Gmail logs in the user and displays the mailbox.
🔹 Step 8-14: User visits YouTube
- From Gmail, the user opens YouTube.
- YouTube sees no login and asks the SSO server.
- The server recognizes the user is already logged in.
- Token is reused and verified.
- YouTube grants access without prompting for credentials again.
That’s the SSO magic in action.
Real-World Use Cases
- Google Workspace: Gmail, YouTube, Google Drive, Docs—all accessible after a single login.
- Microsoft Office 365: Use one Microsoft account to access Word, Excel, Outlook, and Teams.
- Corporate SSO Portals: Enterprises use solutions like Okta, Auth0, or Azure AD to unify logins across platforms.
Practical Example with Code (Using Auth0)
Let’s say you want to integrate SSO using Auth0 in a Node.js application.
1. Setup Auth0 Application
- Go to Auth0 dashboard
- Create a new application
- Set callback URLs, allowed logout URLs
2. Install Required Packages
npm install express express-session passport passport-auth0
3. Basic Setup in app.js
const express = require('express'); const session = require('express-session'); const passport = require('passport'); const Auth0Strategy = require('passport-auth0'); const app = express(); app.use(session({ secret: 'your-session-secret', resave: false, saveUninitialized: true })); passport.use(new Auth0Strategy({ domain: 'YOUR_AUTH0_DOMAIN', clientID: 'YOUR_CLIENT_ID', clientSecret: 'YOUR_CLIENT_SECRET', callbackURL: '/callback' }, function(accessToken, refreshToken, extraParams, profile, done) { return done(null, profile); })); app.use(passport.initialize()); app.use(passport.session()); passport.serializeUser((user, done) => done(null, user)); passport.deserializeUser((user, done) => done(null, user));
4. Add Routes
app.get('/login', passport.authenticate('auth0', { scope: 'openid email profile' })); app.get('/callback', passport.authenticate('auth0', { failureRedirect: '/' }), (req, res) => { res.redirect('/dashboard'); }); app.get('/dashboard', (req, res) => { if (!req.isAuthenticated()) return res.redirect('/login'); res.send(`Hello ${req.user.displayName}`); });
Boom! You now have a simple SSO-enabled login with Auth0.
Challenges in SSO Implementation
While SSO brings a lot of ease, it's not without challenges:
- Complexity in integration
- Token/session management
- Cross-domain authentication issues
- Security risks if not implemented properly
SSO vs OAuth vs OpenID Connect
Often confused, here’s how they differ:
- SSO = One login for multiple apps
- OAuth = Authorization protocol (Who can access what)
- OpenID Connect = Layer on top of OAuth to add identity info
SSO usually uses OAuth/OpenID Connect underneath.
SSO + MFA = Best Practice
You can (and should) combine SSO with Multi-Factor Authentication (MFA) for added security.
SSO is a must-have in any modern application where multiple services or systems are involved. It enhances the user experience while improving security and centralized control.
Have you implemented SSO before? What challenges did you face? Let us know in the comments!
No responses yet