Top 4 Forms of Authentication Mechanisms Explained
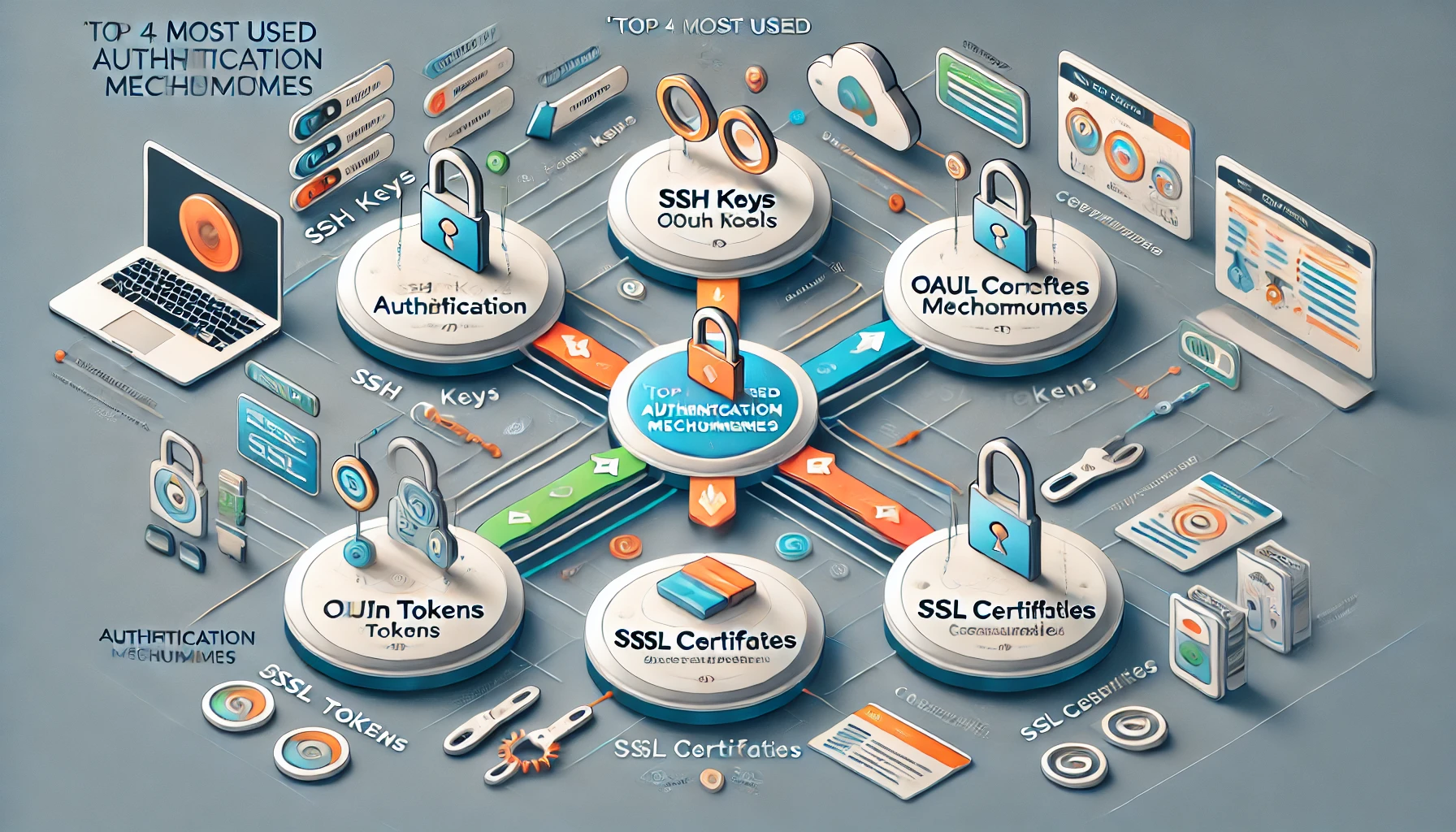
Authentication mechanisms play a critical role in ensuring secure access to systems, data, and applications. Let’s dive deeper into the top four forms of authentication mechanisms, their uses, and how they can be effectively implemented. To enhance understanding, we’ll also include sample code and diagrams where appropriate.
1. SSH Keys
SSH (Secure Shell) keys are cryptographic keys used to securely access remote systems and servers. Unlike traditional passwords, SSH keys offer enhanced security by using a pair of keys: a private key stored securely on the client and a public key installed on the server.
How it works:
- The client sends a request to the server with the public key.
- The server challenges the client to prove possession of the corresponding private key.
- Upon successful verification, access is granted.
Sample Code for SSH Key Authentication:
# Generate an SSH key pair ssh-keygen -t rsa -b 4096 -C "your_email@example.com" # Add the public key to the server ssh-copy-id user@server_address # Securely connect to the server ssh user@server_address
Diagram:
[Client: Private Key] ---- Request + Public Key ----> [Server] [Server] ---- Challenge ----> [Client: Private Key] [Client: Private Key] ---- Response ----> [Server]
2. OAuth Tokens
OAuth (Open Authorization) tokens are used to grant limited access to a user’s data on third-party applications without exposing their credentials. OAuth tokens are often utilized in APIs for authentication and authorization purposes.
How it works:
- A user authorizes a third-party application to access their data.
- The application receives a token that grants limited access to the user’s data.
- Tokens have an expiration time, ensuring limited-time access.
Sample Code for OAuth Token Integration:
// Using Node.js and Express for OAuth token-based authentication const express = require('express'); const axios = require('axios'); const app = express(); const clientID = 'your-client-id'; const clientSecret = 'your-client-secret'; const redirectURI = 'your-redirect-uri'; app.get('/auth/callback', async (req, res) => { const code = req.query.code; const tokenResponse = await axios.post('https://oauth-provider.com/token', { client_id: clientID, client_secret: clientSecret, redirect_uri: redirectURI, code: code, grant_type: 'authorization_code' }); res.send(`Access Token: ${tokenResponse.data.access_token}`); }); app.listen(3000, () => console.log('Server running on port 3000'));
Diagram:
[User] ---- Authorize ----> [Third-Party App] [Third-Party App] ---- Code ----> [OAuth Server] [OAuth Server] ---- Token ----> [Third-Party App]
3. SSL Certificates
SSL (Secure Sockets Layer) certificates ensure secure and encrypted communication between servers and clients. They are essential for protecting sensitive data, such as login credentials and payment information, during transmission.
How it works:
- The server provides its SSL certificate to the client.
- The client verifies the certificate’s validity.
- An encrypted communication channel is established.
Sample Code for SSL Configuration:
# Example NGINX configuration for SSL server { listen 443 ssl; server_name example.com; ssl_certificate /path/to/certificate.crt; ssl_certificate_key /path/to/private.key; location / { proxy_pass http://backend_server; } }
Diagram:
[Client] ---- Request ----> [Server: SSL Certificate] [Server] ---- Validate ----> [Client] [Client] ---- Encrypted Communication ----> [Server]
4. Credentials
Credentials, such as usernames and passwords, are the most common form of authentication. Despite their widespread use, they are also the most vulnerable if not managed properly.
How it works:
- A user provides their credentials.
- The server verifies the credentials against its database.
- Upon successful verification, access is granted.
Sample Code for Credential-Based Authentication:
const express = require('express'); const bcrypt = require('bcrypt'); const app = express(); app.use(express.json()); const users = [ { username: 'user1', password: bcrypt.hashSync('password123', 10) } ]; app.post('/login', (req, res) => { const { username, password } = req.body; const user = users.find(u => u.username === username); if (user && bcrypt.compareSync(password, user.password)) { res.send('Login successful'); } else { res.status(401).send('Invalid credentials'); } }); app.listen(3000, () => console.log('Server running on port 3000'));
Over to You
Managing security keys and credentials is a critical responsibility. Avoid storing sensitive keys and passwords in public repositories like GitHub. Instead, use secure vaults, environment variables, or key management systems to protect them.
How do you manage your security keys? Let us know in the comments below!
1 Comments
Abhinav
This is very useful information Thanks