Mastering RxJS: A Step-by-Step Guide to Reactive Programming in JavaScript
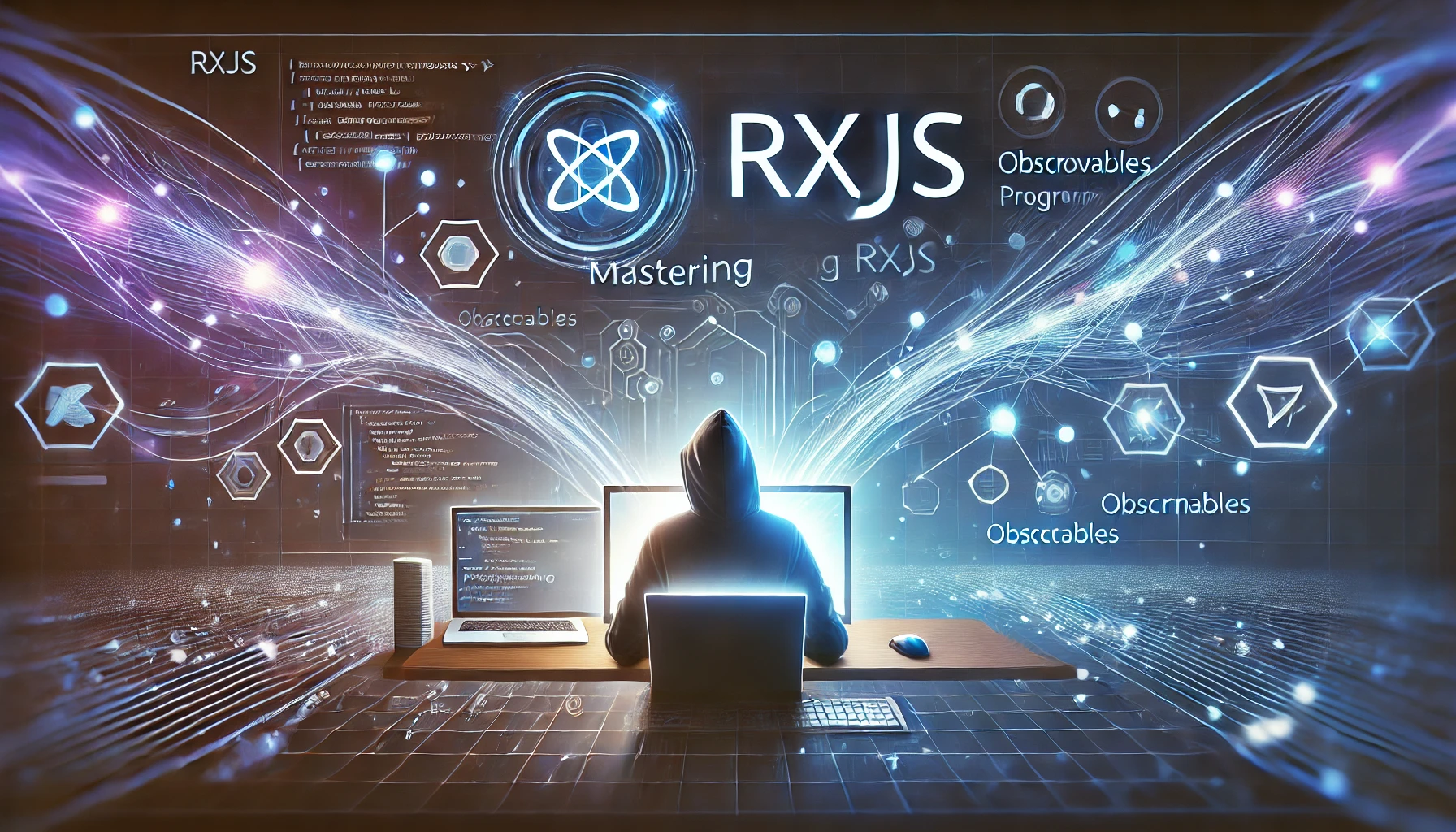
Step 1: Understanding Observables
Reactive programming has revolutionized the way modern developers build applications. At the heart of this paradigm shift is the concept of observables, which allows us to treat all data as a stream that unfolds through the dimension of time. In this tutorial, we'll explore over 25 different ways to leverage the power of RxJS, the Reactive Extensions library for JavaScript, to create robust and scalable applications.
Creating Observables from Scratch
The most fundamental way to create an observable is by using the Rx.Observable.create()
method. This allows us to define the behavior of the observable, such as what values it will emit and when it will complete. We can then subscribe to this observable to receive the emitted values.
Observables from Events and Promises
In addition to creating observables manually, we can also convert other data sources into observables. For example, we can create an observable from DOM events using Rx.Observable.fromEvent()
, or convert a Promise into an observable with Rx.Observable.fromPromise()
. This allows us to seamlessly integrate RxJS into existing JavaScript code.
Observables from Static Data
RxJS also provides a way to create observables from static data, such as arrays, numbers, and objects, using the Rx.Observable.of()
method. This can be useful when you need to represent a fixed set of data as a stream.
Step 2: Understanding Hot and Cold Observables
Observables can be classified as either "hot" or "cold", depending on how they behave when subscribed to. A cold observable is one where the data is created inside the observable itself, meaning that each subscriber will receive a new set of data. In contrast, a hot observable is one where the data is created outside the observable, and all subscribers will receive the same data.
You can convert a cold observable into a hot one by using the publish()
operator, which creates a subject that acts as a proxy for the original observable. This allows you to control when the underlying data is actually created and shared among multiple subscribers.
Step 3: Completing and Unsubscribing from Observables
Observables can be completed, which means they will no longer emit any new values. You can use the finally()
operator to run code when an observable completes. However, some observables, like interval()
, will never complete on their own, which can lead to memory leaks if you don't manually unsubscribe from them.
To manually unsubscribe from an observable, you can store the subscription returned by subscribe()
and call unsubscribe()
on it when you're done. Alternatively, you can use operators like takeUntil()
to automatically complete the observable based on another observable's completion.
Step 4: Transforming Observables with Operators
RxJS provides a wide range of operators that allow you to transform the data emitted by an observable. Here are some of the most commonly used operators:
Map
The map()
operator allows you to transform the emitted values of an observable, for example, by converting a JSON string to a JavaScript object.
Filter
The filter()
operator allows you to selectively emit values from an observable based on a given condition, such as only emitting positive numbers.
Throttle and Debounce
The throttle()
and debounce()
operators are useful for dealing with high-frequency events, such as mouse movements, by limiting the rate at which the observable emits values.
Scan
The scan()
operator is similar to the reduce()
method in JavaScript, allowing you to maintain a running state as the observable emits values. This can be useful for tracking a user's score in a game, for example.
SwitchMap
The switchMap()
operator is particularly useful when you need to create a new observable based on the value emitted by another observable, such as when you need to make an API call based on a user's ID.
Step 5: Combining Observables
RxJS also provides a number of operators for combining multiple observables, such as:
Zip
The zip()
operator combines the latest values from multiple observables into an array, based on their index position.
ForkJoin
The forkJoin()
operator waits for all of the input observables to complete and then emits the last value from each of them as an array.
Step 6: Error Handling and Retrying Observables
Observables can also emit errors, which you can handle using the catch()
operator. This allows you to gracefully handle errors and potentially retry the observable using the retry()
operator.
Step 7: Subjects and Multicasting
RxJS also provides a special type of observable called a "subject", which allows you to both subscribe to and emit values. Subjects can be useful when you need to broadcast data to multiple subscribers without relying on the original data source.
Additionally, you can use the multicast()
operator to create a subject that acts as a proxy for an observable, allowing you to share the underlying data source among multiple subscribers without duplicating any side effects.
By mastering these concepts and techniques, you'll be well on your way to building powerful, reactive applications with RxJS. Remember, the key to success with RxJS is to think in terms of streams of data, rather than individual events or asynchronous operations. With practice and a solid understanding of the core principles, you'll be able to write more efficient, scalable, and maintainable code using this powerful library.
No responses yet