Angular Best Practices for 2024: A Guide for Backend Developers
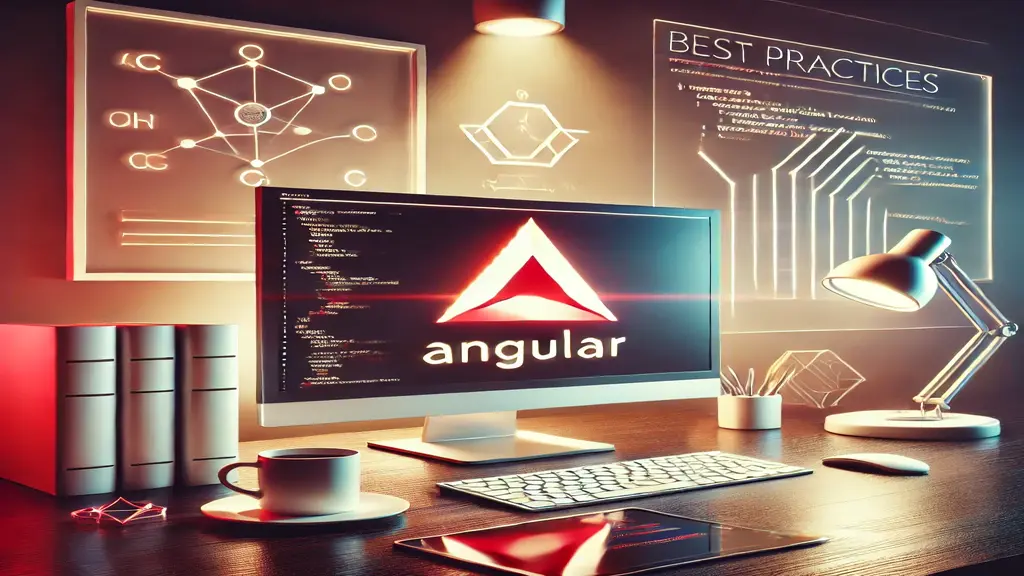
Introduction to Angular
Angular is a TypeScript-based open-source framework maintained by Google. Known for its modular design and powerful tools, Angular is an excellent choice for building large-scale enterprise applications. Backend developers familiar with frameworks like Node.js or Spring often find Angular’s structure intuitive, given its emphasis on separation of concerns and reusable components.
But with great power comes great complexity. To make the most of Angular, developers must follow best practices that enhance:
- Code Quality: Ensuring the application is easy to read and maintain.
- Performance: Optimizing app responsiveness and load times.
- Scalability: Designing for growth, allowing the app to handle increased complexity over time.
Setting Up an Optimal Angular Folder Structure
A well-structured folder system is the foundation of a scalable Angular app. Here’s a recommended structure:
/src /app /core // Singleton services and global utilities /shared // Reusable components, directives, and pipes /features // Feature modules organized by functionality /user /components /services /models user.module.ts /product /components /services /models product.module.ts /assets // Images, styles, icons /environments // Environment-specific configurations
Tips:
- Use feature modules to encapsulate functionality.
- Organize reusable code under shared and global services under core.
- Maintain a clean separation between components, models, and services.
Key Angular Best Practices
Lazy Loading
Lazy loading loads modules only when needed, improving initial load time.
const routes: Routes = [ { path: 'user', loadChildren: () => import('./features/user/user.module').then((m) => m.UserModule), }, ];
Best Practices:
- Apply lazy loading for all feature modules.
- Avoid including unnecessary modules in the initial load.
Naming Conventions
Consistent naming ensures clarity and avoids confusion.
Examples:
- Components: user-profile.component.ts
- Services: auth.service.ts
- Modules: product.module.ts
Best Practices:
- Use PascalCase for class names.
- Use kebab-case for filenames.
Component Communication
Use @Input() and @Output() decorators for parent-child communication.
// Parent Component <app-child [data]="parentData" (event)="handleEvent($event)"></app-child>
Best Practices:
- Avoid tightly coupling components; use services for shared state.
- Consider RxJS Subjects for complex communication.
Readability and Maintainability
Readable code is maintainable code. Focus on clarity over cleverness.
Best Practices:
- Use meaningful variable names.
- Break large components into smaller, focused components.
Dependency Injection
Leverage Angular’s DI system for scalable and testable code.
Example:
@Injectable({ providedIn: 'root', }) export class AuthService { constructor(private http: HttpClient) {} }
Best Practices:
- Use providedIn: 'root' for global services.
- Avoid using new for instantiating dependencies manually.
Routing
Keep your routes clean and well-organized.
const routes: Routes = [ { path: '', redirectTo: '/home', pathMatch: 'full' }, { path: 'home', component: HomeComponent }, { path: '**', component: PageNotFoundComponent }, ];
Best Practices:
- Always include a wildcard route (**) for 404 handling.
- Use route guards for authentication and authorization.
State Management
For complex applications, adopt state management tools like NgRx.
Example:
export const userReducer = createReducer( initialState, on(UserActions.loadUsers, (state) => ({ ...state, loading: true })) );
Best Practices:
- Avoid overusing state management for simple apps.
- Keep actions and reducers modular.
Error Handling
Centralize error handling with interceptors.
Example:
@Injectable() export class ErrorInterceptor implements HttpInterceptor { intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> { return next.handle(req).pipe( catchError((error) => { console.error('Error occurred:', error); return throwError(error); }) ); } }
Performance Optimization
Optimize Angular apps for speed.
Best Practices:
- Use OnPush change detection for components.
- Minimize use of heavy libraries.
- Avoid unnecessary async pipe subscriptions.
Testing
Unit and end-to-end (E2E) testing ensure code quality.
Best Practices:
- Write unit tests for components and services.
- Use tools like Jest or Karma for unit testing.
Security
Protect your application from vulnerabilities.
Best Practices:
- Sanitize inputs to prevent XSS attacks.
- Use Angular’s built-in security features like DomSanitizer.
Documentation
Maintain up-to-date documentation for your app.
Best Practices:
- Use tools like Compodoc for automatic documentation.
- Include clear comments for complex logic.
Deployment
Deploy Angular apps with optimized builds.
Best Practices:
- Use ng build --prod for production builds.
- Configure lazy-loaded routes to minimize initial load.
Accessibility
Ensure your app is accessible to all users.
Best Practices:
- Use ARIA roles and attributes.
- Test with screen readers to ensure compliance.
Code Reviews
Regular code reviews improve code quality and maintainability.
Best Practices:
- Use tools like SonarQube for static code analysis.
- Encourage team collaboration during reviews.
Practical Example: Applying Best Practices in a Sample Project
Let’s implement a simple user management feature that incorporates the best practices discussed.
Folder Structure:
/features/user /components user-list.component.ts user-detail.component.ts /services user.service.ts /models user.model.ts
Sample Code:
User Service:
@Injectable({ providedIn: 'root', }) export class UserService { constructor(private http: HttpClient) {} getUsers(): Observable<User[]> { return this.http.get<User[]>('/api/users'); } }
User List Component:
@Component({ selector: 'app-user-list', template: ` <div *ngFor="let user of users"> {{ user.name }} </div> `, }) export class UserListComponent implements OnInit { users: User[] = []; constructor(private userService: UserService) {} ngOnInit() { this.userService.getUsers().subscribe((data) => (this.users = data)); } }
This simple example highlights how adhering to best practices improves code clarity and modularity.
Conclusion and Call-to-Action
By implementing these Angular best practices for 2024, you can build scalable, maintainable, and high-performing applications. Whether you’re optimizing performance, streamlining state management, or ensuring accessibility, these strategies will set your projects up for success.
1 Comments
Chaya
very informative angular blog